- To use the Dynamic Reconfigure utility, we will write a configuration file and save it in the cfg folder in the intended package.
- Create the cfg folder and a parameter_server_tutorials.cfg file there in the parameter_server_tutorials package, as follows:
$ roscd parameter_server_tutorials $ mkdir cfg $ vim parameter_server_tutorials
- We will add the following code in the parameter_server_tutorials.cfg file:
#!/usr/bin/env python PACKAGE = "parameter_server_tutorials" from dynamic_reconfigure.parameter_generator_catkin import * gen = ParameterGenerator() gen.add("BOOL_PARAM", bool_t, 0,"A Boolean parameter", True) gen.add("INT_PARAM", int_t, 0, "An Integer Parameter", 1, 0, 100) gen.add("DOUBLE_PARAM", double_t, 0, "A Double Parameter", 0.01, 0, 1) gen.add("STR_PARAM", str_t, 0, "A String parameter", "Dynamic Reconfigure") size_enum = gen.enum([ gen.const("Low", int_t, 0, "Low : 0"), gen.const("Medium", int_t, 1,"Medium : 1"), gen.const("High", int_t, 2, "Hight :2")], "Selection List") gen.add("SIZE", int_t, 0, "Selection List", 1, 0, 3, edit_method=size_enum) exit(gen.generate(PACKAGE, "parameter_server_tutorials", "parameter_server_"))
- To initialize the parameter generator and add parameters in the following few lines, add the following:
gen = ParameterGenerator() gen.add("BOOL_PARAM", bool_t, 0,"A Boolean parameter", True) gen.add("INT_PARAM", int_t, 0, "An Integer Parameter", 1, 0, 100) gen.add("DOUBLE_PARAM", double_t, 0, "A Double Parameter", 0.01, 0, 1) gen.add("STR_PARAM", str_t, 0, "A String parameter", "Dynamic Reconfigure") size_enum = gen.enum([gen.const("Low", int_t, 0, "Low : 0"), gen.const("Medium", int_t, 1, "Medium : 1"), gen.const("High", int_t, 2, "Hight :2")],"Selection List") gen.add("SIZE", int_t, 0, "Selection List", 1, 0, 3, edit_method=size_enum)
- These lines add different parameter types and set the default values, description, range, and so on. Moreover, the parameter has the following arguments:
gen.add(name, type, level, description, default, min, max)
The arguments are defined as follows:
-
- name: The name of the parameter
- type: The type of value stored
- level: The bitmask that is passed to the callback
- description: The description of the parameter
- default: The default value when the node starts
- min: The minimum value for the parameter
- max: The maximum value for the parameter
Finally, the last line of the previous code generates the necessary files and exits the program. Here, we can see that the .cfg file is written in Python. However, this book is for C++, but sometimes we will use Python whenever it is appropriate and it is required to explain a certain concept:
exit(gen.generate(PACKAGE, "parameter_server_tutorials", "parameter_server_"))
It is necessary to change the permissions for the parameter_server_tutorials.cfg file because the file will be executed by ROS as Python scripts:
$ chmod a+x cfg/ parameter_server_tutorials.cfg
- For invoking the compilation, the following lines should be added in CMakeList.txt :
find_package(catkin REQUIRED COMPONENTS roscpp std_msgs message_generation dynamic_reconfigure ) generate_dynamic_reconfigure_options( cfg/parameter_server_tutorials.cfg ) add_dependencies(parameter_server_tutorials parameter_server_tutorials_gencfg)
- Create an example node with the Dynamic Reconfigure support:
$ roscd parameter_server_tutorials $ vim src/ parameter_server_tutorials.cpp
We will add the following code snippet in the node file:
#include <ros/ros.h> #include <dynamic_reconfigure/server.h> #include <parameter_server_tutorials/parameter_server_Config.h> void callback(parameter_server_tutorials::parameter_server_Config &config, uint32_t level) { ROS_INFO("Reconfigure Request: %s %d %f %s %d", config.BOOL_PARAM?"True":"False", config.INT_PARAM, config.DOUBLE_PARAM, config.STR_PARAM.c_str(), config.SIZE); } int main(int argc, char **argv) { ros::init(argc, argv, "parameter_server_tutorials"); dynamic_reconfigure::Server<parameter_server_tutorials::parameter_server_Config> server; dynamic_reconfigure::Server<parameter_server_tutorials::parameter_server_Config>::CallbackType f; f = boost::bind(&callback, _1, _2); server.setCallback(f); ROS_INFO("Spinning"); ros::spin(); return 0; }
As usual, these lines include the headers for ROS, the parameter server, and the config file that we created earlier:
#include <ros/ros.h> #include <dynamic_reconfigure/server.h> #include <parameter_server_tutorials/parameter_server_Config.h>
The following lines of code show the callback function, which will print the values for the parameters that are modified by the user. Notice that the name of the parameter must be the same as the one that is configured in the parameter_server_tutorials.cfg file:
void callback(parameter_server_tutorials::parameter_server_Config &config, uint32_t level) { ROS_INFO("Reconfigure Request: %s %d %f %s %d", config.BOOL_PARAM?"True":"False", config.INT_PARAM, config.DOUBLE_PARAM, config.STR_PARAM.c_str(), config.SIZE); }
Moreover, in the main function, the server is initialized with the parameter_server_Config configuration file. The next callback function is set to the server which will be called, which is when the server gets a reconfiguration request:
dynamic_reconfigure::Server<parameter_server_tutorials::parameter_server_Config> server; dynamic_reconfigure::Server<parameter_server_tutorials::parameter_server_Config>::CallbackType f; f = boost::bind(&callback, _1, _2); server.setCallback(f);
- Finally, we have to add the following lines to the CMakeLists.txt file for the ROS build system to invoke the compilation:
add_executable(parameter_server_tutorials src/parameter_server_tutorials.cpp) add_dependencies(parameter_server_tutorials parameter_server_tutorials_gencfg) target_link_libraries(parameter_server_tutorials ${catkin_LIBRARIES}
Great! We are done with the development part. Now, we have to compile and run the node and the Dynamic Reconfigure GUI as follows:
$ roscore $ rosrun parameter_server_tutorials parameter_server_tutorials $ rosrun rqt_reconfigure rqt_reconfigure
- To dynamically modify the parameters of the node, as shown in the following screenshot:
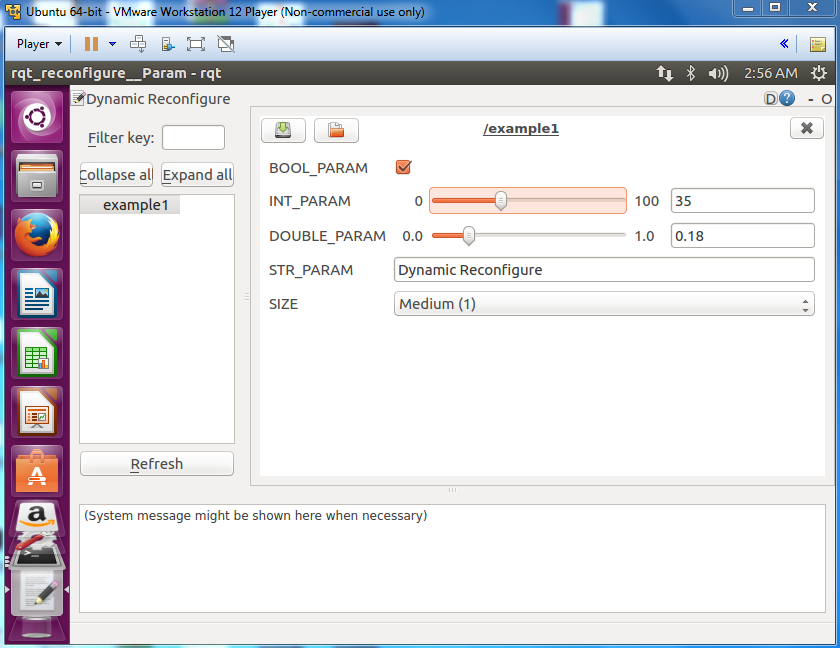
Each time a user modifies a parameter with the slider, the checkbox, and so on, we will observe the changes made in the shell where the node is running:

Thanks to the Dynamic Reconfigure utility of ROS, the node which interfaces with the hardware can be tuned and validated more efficiently and faster. We will learn more about this in the upcoming chapters.