PDF files are a slightly difficult task; since Unity doesn't open them internally, an external application must be used. In this section, we are going to learn about a simple call that we can use for opening the PDF files but that can also be used for other types of extensions (such as videos) and opening server files through a URL.
As you may remember, we have not placed the video and PDF files inside the @MyAssets folder but in the StreamingAssets/PDF folder. This folder is a special folder inside Unity, and all the files in it are copied verbatim to the destination device, meaning they are not processed by Unity at all. We can't load them directly from this path, so we will copy them to an accessible path first.
Go to Visual Studio and in the MainHandler.cs script, let's add some code to handle these files. Follow these steps to do so:
- Add the System.IO library:
using System.IO;
- Add the following variables at the beginning to indicate the paths of the PDF file inside the device:
private string originalPath;
private string savePath;
- Initialize them inside the Start() method:
originalPath = Application.streamingAssetsPath + "/PDF/WorkOrder_0021.pdf";
savePath = Application.persistentDataPath + "/WorkOrder_0021.pdf";
- Add the following coroutine, which copies the PDF file from the StreamingAssets location to an accessible path and opens it:
private IEnumerator OpenFileCoroutine()
{
WWW www = new WWW(originalPath);
yield return www;
if (www.error != null)
Debug.Log("Error loading: " + www.error);
else
{
byte[] bytes = www.bytes;
File.WriteAllBytes(savePath, bytes);
Application.OpenURL(savePath);
}
}
- Finally, add the following public method to open the PDF file:
public void OpenPDFFile()
{
if (File.Exists(savePath))
Application.OpenURL(savePath);
else
StartCoroutine(OpenFileCoroutine());
}
If the file already exists in an accessible location, it opens it. Otherwise, it copies first and opens it from the coroutine.
Back in Unity editor, select the File_button, and in the Inspector window, add the OpenPDFfile() call to its On Click () event:
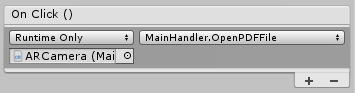
Press Ctrl + B one last time to see the full app in the glasses.