Before looking at the assembly instruction to move a value from the memory to a register, let's try to understand how values reside in the memory. Let's say you have defined a variable in your C program:
int val = 100;
The following list outlines what happens during the runtime of the program:
- An integer is 4 bytes in length, so the integer 100 is stored as a sequence of 4 bytes (00 00 00 64) in the memory.
- The sequence of four bytes is stored in the little-endian format mentioned previously.
- The integer 100 is stored at some memory address. Let's assume that 100 was stored at the memory address starting at 0x403000; you can think of this memory address labeled as val:
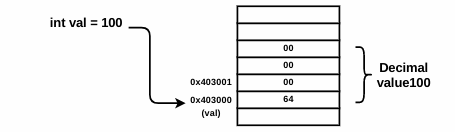
To move a value from the memory into a register in assembly language, you must use the address of the value. The following assembly instruction will move the 4 bytes stored at the memory address 0x403000 into the register eax. The square bracket specifies that you want the value stored at the memory location, rather than the address itself:
mov eax,[0x403000] ; eax will now contain 00 00 00 64 (i.e 100)
Notice that in the preceding instruction, you did not have to specify 4 bytes in the instruction; based on the size of the destination register (eax), it automatically determined how many bytes to move. The following screenshot will help you to understand what happens after executing the preceding instruction:

During reverse engineering, you will normally see instructions similar to the ones shown as below. The square brackets may contain a register, a constant added to a register, or a register added to a register. All of the following diagram instructions move values stored at the memory address specified within the square brackets to the register. The simplest thing to remember is that everything within the square brackets represents an address:
mov eax,[ebx] ; moves value at address specifed by ebx register
mov eax,[ebx+ecx] ; moves value at address specified by ebx+ecx
mov ebx,[ebp-4] ; moves value at address specified by ebp-4
Another instruction that you will normally come across is the lea instruction, which stands for load effective address; this instruction will load the address instead of the value:
lea ebx,[0x403000] ; loads the address 0x403000 into ebx
lea eax, [ebx] ; if ebx = 0x403000, then eax will also contain 0x403000
Sometimes, you will come across instructions like the ones that follow. These instructions are the same as the previously mentioned instructions and transfer data stored in a memory address (specified by ebp-4) into the register. The dword ptr just indicates that a 4-byte (dword) value is moved from the memory address specified by ebp-4 into the eax:
mov eax,dword ptr [ebp-4] ; same as mov eax,[ebp-4]