We are sure that you have been playing with the robot by moving it around the map a lot. This is funny but a little tedious, and it is not very functional.
Perhaps you were thinking that it would be a great idea to program a list of movements and send the robot to different positions with only a button, even when we are not in front of a computer with rviz.
Okay, now you are going to learn how to do it using actionlib.
The actionlib package provides a standardized interface for interfacing with tasks. For example, you can use it to send goals for the robot to detect something at a place, make scans with the laser, and so on. In this section, we will send a goal to the robot, and we will wait for this task to end.
It could look similar to services, but if you are doing a task that has a long duration, you might want the ability to cancel the request during the execution, or get periodic feedback about how the request is progressing. You cannot do this with services. Furthermore, actionlib creates messages (not services), and it also creates topics, so we can still send the goals through a topic without taking care of the feedback and the result, if we do not want to.
The following code is a simple example for sending a goal to move the robot. Create a new file in the chapter6_tutorials/src folder, and add the following code in a file with the name sendGoals.cpp:
#include <ros/ros.h> #include <move_base_msgs/MoveBaseAction.h> #include <actionlib/client/simple_action_client.h> #include <tf/transform_broadcaster.h> #include <sstream> typedefactionlib::SimpleActionClient<move_base_msgs::
MoveBaseAction>MoveBaseClient; int main(int argc, char** argv){ ros::init(argc, argv, "navigation_goals"); MoveBaseClientac("move_base", true); while(!ac.waitForServer(ros::Duration(5.0))){ ROS_INFO("Waiting for the move_base action server"); } move_base_msgs::MoveBaseGoal goal; goal.target_pose.header.frame_id = "map"; goal.target_pose.header.stamp = ros::Time::now();
goal.target_pose.pose.position.x = 1.0; goal.target_pose.pose.position.y = 1.0; goal.target_pose.pose.orientation.w = 1.0; ROS_INFO("Sending goal"); ac.sendGoal(goal); ac.waitForResult(); if(ac.getState() == actionlib::SimpleClientGoalState::SUCCEEDED) ROS_INFO("You have arrived to the goal position"); else{ ROS_INFO("The base failed for some reason"); } return 0; }
Add the next file in the CMakeList.txt file to generate the executable for our program:
add_executable(sendGoalssrc/sendGoals.cpp) target_link_libraries(sendGoals ${catkin_LIBRARIES})
Now compile the package with the following command:
$ catkin_make
Now launch everything to test the new program. Use the following commands to launch all the nodes and the configurations:
$ roslaunch chapter6_tutorials chapter6_configuration_gazebo.launch $ roslaunch chapter6_tutorials move_base.launch
Once you have configured the 2D pose estimate, run the sendGoal node with the next command in a new shell:
$ rosrun chapter6_tutorials sendGoals
If you go to the rviz screen, you will see a new global plan (green line) over the map. This means that the navigation stack has accepted the new goal and it will start to execute it.
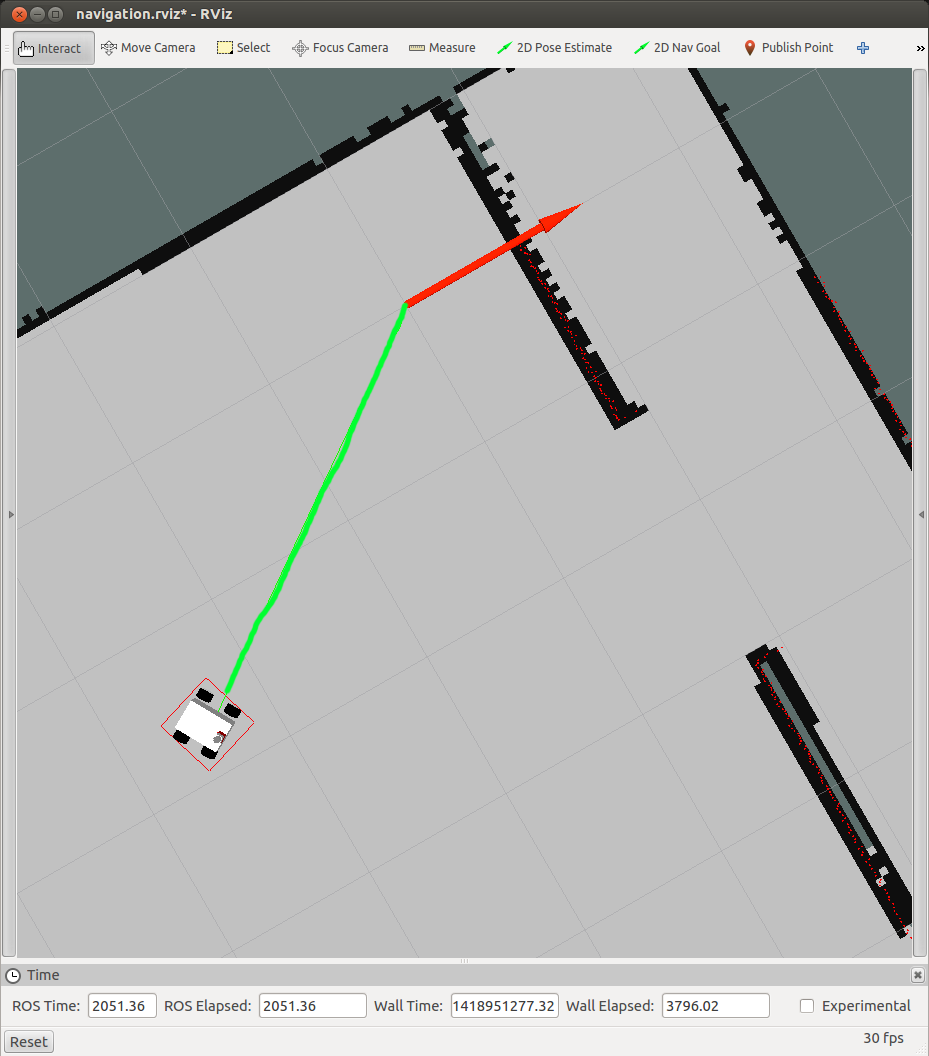
When the robot arrives at the goal, you will see the following message in the shell where you ran the node:
[ INFO ] [...,...]: You have arrived to the goal position
You can make a list of goals or waypoints, and create a route for the robot. This way you can program missions, guardian robots, or collect things from other rooms with your robot.