Now we will create a new file in chapter5_tutorials/src with the name laser.cpp and put the following code in it:
#include <ros/ros.h> #include <sensor_msgs/LaserScan.h> int main(int argc, char** argv){ ros::init(argc, argv, "laser_scan_publisher"); ros::NodeHandle n; ros::Publisher scan_pub = n.advertise<sensor_
msgs::LaserScan>("scan", 50); unsigned int num_readings = 100; double laser_frequency = 40; double ranges[num_readings]; double intensities[num_readings]; int count = 0; ros::Rate r(1.0); while(n.ok()){ //generate some fake data for our laser scan for(unsigned int i = 0; i < num_readings; ++i){ ranges[i] = count; intensities[i] = 100 + count; } ros::Time scan_time = ros::Time::now(); //populate the LaserScan message sensor_msgs::LaserScan scan; scan.header.stamp = scan_time; scan.header.frame_id = "base_link"; scan.angle_min = -1.57; scan.angle_max = 1.57; scan.angle_increment = 3.14 / num_readings; scan.time_increment = (1 / laser_frequency) / (num_readings); scan.range_min = 0.0; scan.range_max = 100.0; scan.ranges.resize(num_readings); scan.intensities.resize(num_readings); for(unsigned int i = 0; i < num_readings; ++i){ scan.ranges[i] = ranges[i]; scan.intensities[i] = intensities[i]; } scan_pub.publish(scan); ++count; r.sleep(); } }
As you can see, we are going to create a new topic with the name scan and the message type sensor_msgs/LaserScan. You will become familiar with this message type when you reach Chapter 7, Using Sensors and Actuators with ROS. The name of the topic must be unique. When you configure the navigation stack, you will select this topic to be used for navigation. The following command line shows how to create the topic with the correct name:
ros::Publisher scan_pub =
n.advertise<sensor_msgs::LaserScan>("scan", 50);
It is important to publish data with header, stamp, frame_id, and many more elements because, if not, the navigation stack could fail with such data:
scan.header.stamp = scan_time; scan.header.frame_id = "base_link";
Other important data on header is frame_id. It must be one of the frames created in the .urdf file and must have a frame published on the tf frame transforms. The navigation stack will use this information to know the real position of the sensor and make transforms, such as the one between the data sensor and obstacles.
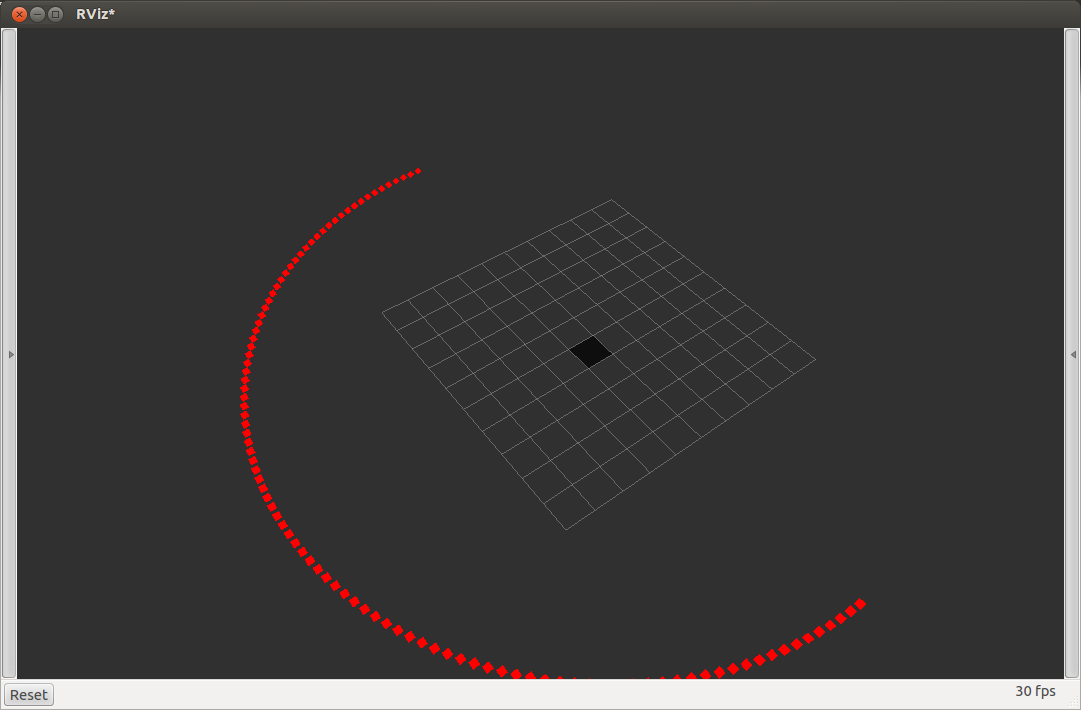
With this template, you can use any laser, even if it has no driver for ROS. You only have to change the fake data with the right data from your laser.
This template can also be used to create something that looks like a laser but is not. For example, you could simulate a laser using stereoscopy or using a sensor such as a sonar.