Let's start coding using TensorFlow. We are again going to write basic code that performs matrix operations such as matrix addition, multiplication, scalar multiplication and multiplication with a scalar from 1 to 99. The code is written for demonstrating basic capabilities of TensorFlow, which we have discussed previously.
Here is the code for all these operations:
import tensorflow as tf import time matrix_1 = tf.Variable([[1,2,3],[4,5,6],[7,8,9]],name="mat1") matrix_2 = tf.Variable([[1,2,3],[4,5,6],[7,8,9]],name="mat2") scalar = tf.constant(5) number = tf.Variable(1, name="counter") add_msg = tf.constant(" Result of matrix addition ") mul_msg = tf.constant(" Result of matrix multiplication ") scalar_mul_msg = tf.constant(" Result of scalar multiplication ") number_mul_msg = tf.constant(" Result of Number multiplication ") mat_add = tf.add(matrix_1,matrix_2) mat_mul = tf.matmul(matrix_1,matrix_2) mat_scalar_mul = tf.mul(scalar,mat_mul) mat_number_mul = tf.mul(number,mat_mul) init_op = tf.initialize_all_variables() sess = tf.Session() tf.device("/cpu:0") sess.run(init_op) for i in range(1,100): print " For i =",i print(sess.run(add_msg)) print(sess.run(mat_add)) print(sess.run(mul_msg)) print(sess.run(mat_mul)) print(sess.run(scalar_mul_msg)) print(sess.run(mat_scalar_mul)) update = tf.assign(number,tf.constant(i)) sess.run(update) print(sess.run(number_mul_msg)) print(sess.run(mat_number_mul)) time.sleep(0.1) sess.close()
As we know, we have to import the tensorflow module to access its APIs. We are also importing the time module to provide a delay in the loop:
import tensorflow as tf import time
Here is how to define variables in TensorFlow. We are defining matrix_1 and matrix_2 variables, two 3x3 matrices:
matrix_1 = tf.Variable([[1,2,3],[4,5,6],[7,8,9]],name="mat1") matrix_2 = tf.Variable([[1,2,3],[4,5,6],[7,8,9]],name="mat2")
In addition to the preceding matrix variables, we are defining a constant and a scalar variable called counter. These values are used for scalar multiplication operations. We will change the value of counter from 1 to 99, and each value will be multiplied with a matrix:
scalar = tf.constant(5) number = tf.Variable(1, name="counter")
The following is how we define strings in TF. Each string is defined as a constant.
add_msg = tf.constant(" Result of matrix addition ") mul_msg = tf.constant(" Result of matrix multiplication ") scalar_mul_msg = tf.constant(" Result of scalar multiplication ") number_mul_msg = tf.constant(" Result of Number multiplication ")
The following are the main ops in the graph doing the computation. The first line will add two matrices, second line will multiply those same two, the third will perform scalar multiplication with one value, and the fourth will perform scalar multiplication with a scalar variable.
mat_add = tf.add(matrix_1,matrix_2) mat_mul = tf.matmul(matrix_1,matrix_2) mat_scalar_mul = tf.mul(scalar,mat_mul) mat_number_mul = tf.mul(number,mat_mul)
If we have TF variable declarations, we have to initialize them using the following line of code:
init_op = tf.initialize_all_variables()
Here, we are creating a Session() object:
sess = tf.Session()
This is one thing we hadn't discussed earlier. We can perform the computation on any device according to our priority. It can be a CPU or GPU. Here, you can see that the device is a CPU:
tf.device("/cpu:0")
This line of code will run the graph to initialize all variables:
sess.run(init_op)
In the following loop, we can see the running of a TF graph. This loop puts each op inside the run() method and fetches its results. To be able to see each output, we are putting a delay on the loop:
for i in range(1,100): print " For i =",i print(sess.run(add_msg)) print(sess.run(mat_add)) print(sess.run(mul_msg)) print(sess.run(mat_mul)) print(sess.run(scalar_mul_msg)) print(sess.run(mat_scalar_mul)) update = tf.assign(number,tf.constant(i)) sess.run(update) print(sess.run(number_mul_msg)) print(sess.run(mat_number_mul)) time.sleep(0.1)
After all this computation, we have to release the Session() object to free up the resources:
sess.close()
The following is the output:
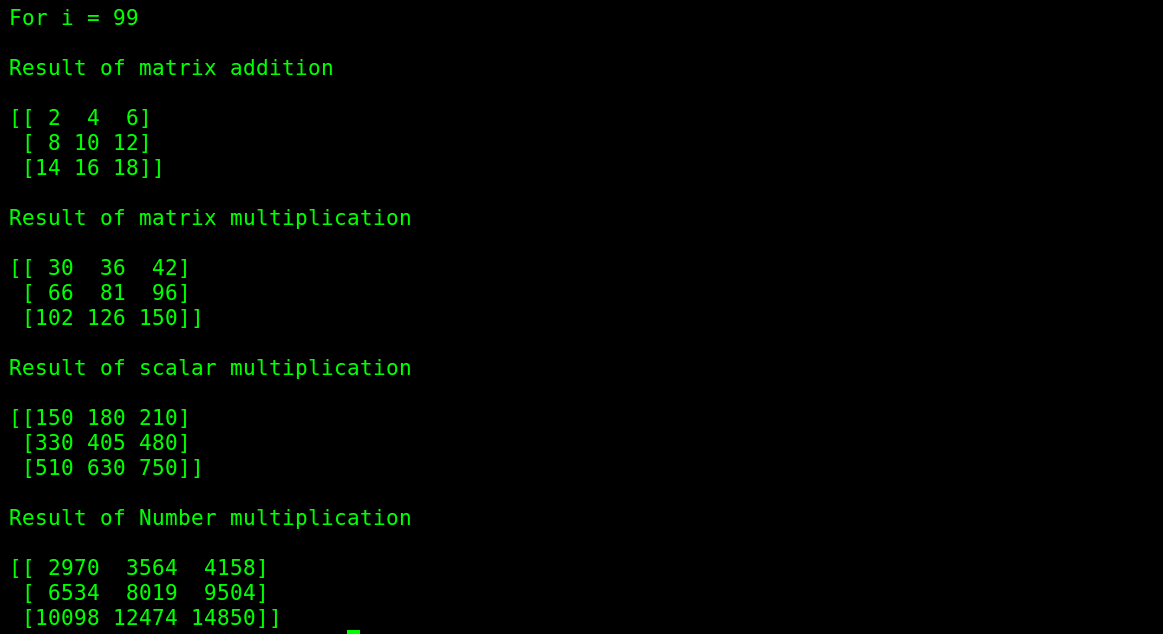