PCL provides several ways of visualizing point clouds. The first and simplest is through the basic cloud viewer, which is capable of representing any sort of PCL point cloud in a 3D viewer, while at the same time providing a set of callbacks for user interaction. In the following example, we will create a small node that will subscribe to sensor_msgs/PointCloud2 and the node will display sensor_msgs/PointCloud2 using cloud_viewer (basic) from the library. The code for this example can be found in the chapter10_tutorials/src source directory, and it is named pcl_visualize.cpp:
#include <iostream> #include <ros/ros.h> #include <pcl/visualization/cloud_viewer.h> #include <sensor_msgs/PointCloud2.h> #include <pcl_conversions/pcl_conversions.h> class cloudHandler { public: cloudHandler() : viewer("Cloud Viewer") { pcl_sub = nh.subscribe("pcl_output", 10,
&cloudHandler::cloudCB, this); viewer_timer = nh.createTimer(ros::Duration(0.1),
&cloudHandler::timerCB, this); } void cloudCB(const sensor_msgs::PointCloud2 &input) { pcl::PointCloud<pcl::PointXYZ> cloud; pcl::fromROSMsg(input, cloud); viewer.showCloud(cloud.makeShared()); } void timerCB(const ros::TimerEvent&) { if (viewer.wasStopped()) { ros::shutdown(); } } protected: ros::NodeHandle nh; ros::Subscriber pcl_sub; pcl::visualization::CloudViewer viewer; ros::Timer viewer_timer; }; main (int argc, char **argv) { ros::init (argc, argv, "pcl_visualize"); cloudHandler handler; ros::spin(); return 0; }
The code for this particular example introduces a different pattern; in this case, all of our functionality is encapsulated in a class, which provides a clean way of sharing variables with the callback functions, as opposed to using global variables.
The constructor implicitly initializes the node handle through the default constructor, which is automatically called for the missing objects in the initializer list. The cloud handle is explicitly initialized with a very simple string, which corresponds to the window name after everything is correctly initialized. The subscriber to the pcl_output topic is set as well as a timer, which will trigger a callback every 100 milliseconds. This timer is used to periodically check whether the window has been closed and, if this is the case, shut down the node:
cloudHandler() : viewer("Cloud Viewer") { pcl_sub = nh.subscribe("pcl_output", 10, &cloudHandler::cloudCB,
this); viewer_timer = nh.createTimer(ros::Duration(0.1),
&cloudHandler::timerCB, this); }
The point cloud callback function is not very different from the previous examples except that, in this particular case, the PCL point cloud is passed directly to the viewer through the showCloud function, which automatically updates the display:
void cloudCB(const sensor_msgs::PointCloud2 &input) { pcl::PointCloud<pcl::PointXYZ> cloud; pcl::fromROSMsg(input, cloud); viewer.showCloud(cloud.makeShared()); }
Since the viewer window usually comes with a close button as well as a keyboard shortcut to close the window, it is important to take into account this event and act upon it by, for example, shutting down the node. In this particular case, we are handling the current state of the window in a callback, which is called through a ROS timer every 100 milliseconds. If the viewer has been closed, our action is to simply shut down the node:
void timerCB(const ros::TimerEvent&) { if (viewer.wasStopped()) { ros::shutdown(); } }
To execute this example, and any other for that matter, the first step is to run the roscore command in a terminal:
$ roscore
In a second terminal, we will run the pcl_read example and a source of data, such as a reminder, using the following commands:
$ roscd chapter10_tutorials/data $ rosrun chapter10_tutorials pcl_read
Finally, in a third terminal, we will run the following command:
$ rosrun chapter10_tutorials pcl_visualize
Running this code will cause a window to launch; this window will display the point cloud contained in the test PCD file provided with the examples. The following screenshot shows this:
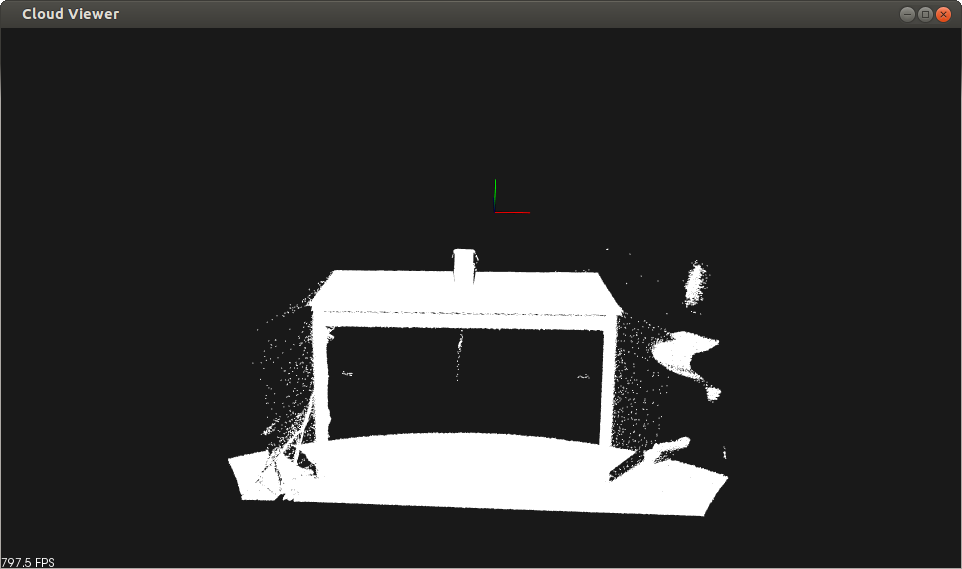
The current example uses the simplest possible viewer, namely the PCL cloud_viewer, but the library also provides a much more complex and complete visualization component named PCLVisualizer. This visualizer is capable of displaying point clouds, meshes, and surfaces, as well as including multiple viewports and color spaces. An example of how to use this particular visualizer is provided in the chapter10_tutorials source directory named pcl_visualize2.cpp.
In general, all the visualizers provided by PCL use the same underlying functionality and work in much the same way. The mouse can be used to move around the 3D view; in combination with the shift, it allows you to translate the image, and in combination with the control, it allows you to rotate the image. Finally, upon pressing H, the help text is printed in the current terminal, which should look like the following screenshot:
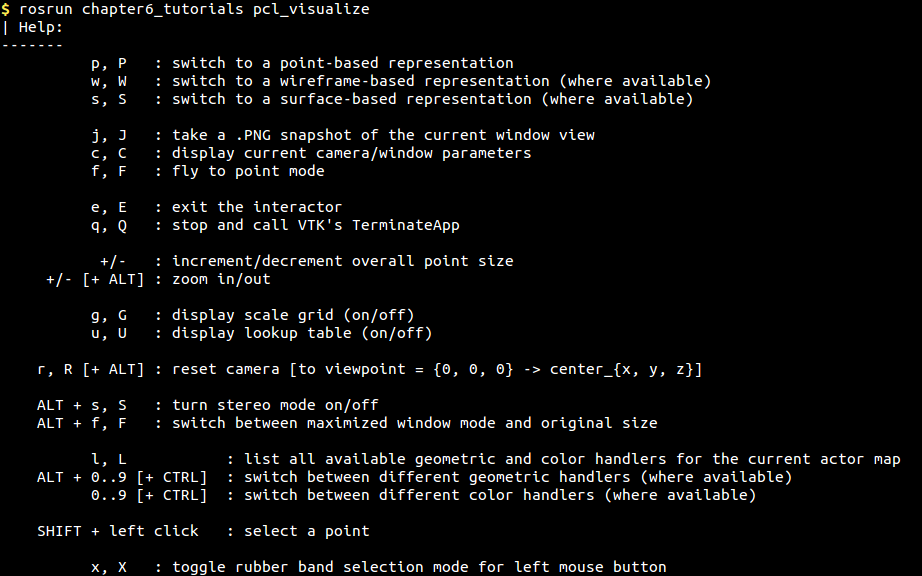